PHP is a recursive acronym that stands for PHP: Hypertext Preprocessor; this is in the naming style of GNU, which stands for GNU’s Not Unix and which began this odd trend. The name isn’t a particularly good description of what PHP is and what it’s commonly used for. This tutorial covers the following topics:
- PHP Basics
- PHP Tags
- PHP Comments
- Output data – echo and print
- Shortcut – An alternate to print or echo
- print vs echo
A PHP script can perform the following tasks:
- Process Web forms sent by the visitors
- Read, write, and create files on the Web server
- Insert, retrieve and edit data in a database stored on the Web server
- Generate charts and manipulate images
Why Use PHP?
- A large number of Web hosting companies support it
- Thousands of developers are using PHP
- A million sites have PHP installed
- Cross-platform, — a PHP script can run on Windows, Linux, Mac OS, and many others
- Can integrate with common Web servers, such as Apache, IIS, lighttpd, Nginx, etc.
PHP Basics
PHP has its roots in C
and was created to be a more accessible language to make web-pages in than C
. All statements in PHP must be terminated with a semicolon. An exception to this is if the statement happens to be the last statement before a closing tag. Coding standards would normally require you to properly terminate every statement.
Whitespace has no semantic meaning in PHP. There is no need to line code up, but most coding standards enforce this to improve code readability. Whitespace may not appear in the middle of function names, variable names, or keywords. Multiple statements are allowed on a single line.
Code blocks are denoted by the brace symbols { }
.
PHP Tags
PHP is a text processor to support webpages. When PHP parses a file, it looks for opening and closing tags, which are <?php
and ?>
which tell PHP to start and stop interpreting the code between them. Parsing in this manner allows PHP to be embedded in all sorts of different documents, as everything outside of a pair of opening and closing tags is ignored by the PHP parser.
PHP supports the following five tags:
Opening tag | Closing tag | |
---|---|---|
<?php | ?> | Standard tag (recommended) |
<?= | ?> | Echo tag (Always available since PHP 5.4.0) |
<? | ?> | Short tag (only available if enabled in php.ini file). |
<% | %> | ASP tag (removed since PHP 7.0.0.) |
<script language="php"> | </script> | Script tag (removed since PHP 7.0.0) |
The echo tag allows you to easily echo a PHP variable and the shortened tag makes your HTML document easier to read. Its usage is easiest to understand when it’s shown along with the equivalent in standard opening codes. The following two tags are identical:
<?= $variable ?> <?php echo $variable ?>
Don’t write the PHP closing tag
It is quite common in PHP programs to omit the closing tag ?>
in a file. This is acceptable to the parser and is a useful way to prevent problems with newline characters appearing after the closing tag. These new line characters would be sent as output by the PHP interpreter and could interfere with the HTTP headers or cause other unintended side effects. By not closing the script in a PHP file you prevent the chance of newline characters being sent.
Example 1 shows the first PHP script in this tutorial, the ubiquitous “Hello, world”. When requested by a web browser, the script is run on the webserver, and the resulting HTML document is sent back to the browser and rendered as shown in the following figure:
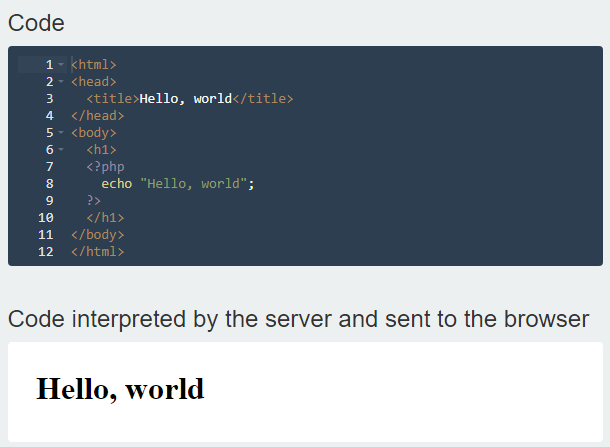
Example 1. The ubiquitous Hello, world in PHP:
<html> <head> <title>Hello, world</title> </head> <body> <h1> <?php echo "Hello, world"; ?> </h1> </body> </html>
Example 1 illustrates the basic features of a PHP script. It’s a mixture of HTML, in this case, it’s mostly HTML and a PHP script. The PHP in this example simply prints the greeting, “Hello, world.”:
<?php echo "Hello, world"; ?>
The PHP script shown in Example-1 is rather pointless: we could simply have authored the HTML to include the greeting directly. Because PHP integrates so well with HTML, using PHP to produce static strings is far less complicated and less interesting than using other high-level languages. However, the example does illustrate several features of PHP:
- The begin and end script tags are
<?php
and?>
or, if enabled in the php.ini file, just<?
and?>
. The longer beginning tag style<?php
avoids conflicts with other processing instructions that can be used in HTML. - Whitespace has no effect, except to aid readability for the developer. For example, the script could have been written succinctly as
<?php echo "Hello,
world";?>
with the same effect. Any mix of spaces, tabs, carriage returns, and so on in separating statements is allowed. - A PHP script is a series of statements, each terminated with a semicolon. Our simple example has only one statement:
echo "Hello, world";
. - A PHP script can be anywhere in a file and interleaved with any HTML fragment. While Example 1 contains only one script, there can be any number of PHP scripts in a file.
- When a PHP script is run, the entire script including the start and end script tags
<?php
and?>
is replaced with the output of the script.
The freedom to interleave any number of scripts with HTML is one of the most powerful features of PHP. A short example is shown in Example 2; a variable, $output="Hello,world"
, is initialized before the start of the HTML document, and later this string variable is output twice, as part of the <title>
and <body>
elements. We discuss more about variables and how to use them later in this tutorial.
Example 2. Embedding three scripts in a single document
<!--You can ignore the semicolon for last statement before a closing tag--> <?php $output = "Hello, world" ?> <html> <head> <title><?php echo $output; ?></title> </head> <body> <!--Using echo short tag--> <h1><?= $output ?></h1> </body> </html>
The flexibility to add multiple PHP codes to HTML can also lead to unwieldy, hard-to-maintain code. Care should be taken in modularizing code and HTML; we discuss how to separate code and HTML using templates later.
PHP Comments
Comments can be included in code using familiar styles from other high-level programming languages. This includes the following styles:
// This is a one-line comment # This is another one-line comment style /* This is how you can create a multi-line comment */
Single line | Multi line | |
---|---|---|
Perl style | # | |
C style | // | /* Multiline */ |
API style | /** * API doc style */ |
Output data – echo and print commands
The echo
statement is frequently used and designed to output any type of data. In most cases, anything
output by echo
ends up viewable in the web browser. The print
statement can be used for the same purpose. Consider some examples:
echo "Hello, world"; // print works just the same print "Hello, world"; // numbers can be printed too echo 123; // So can the contents of variables $outputString = 'Some Text'; echo $outputString;
PHP Shortcut to Print (or echo) data
There is also a shortcut that can output data: <?=
tag. You can use it like so: <?="Hello world"?>
. It known as short tag. In PHP 7, alternative PHP tags, <%
, <%=
, %>
and <script language="php">
, have been removed, but the short opening tags <?
(which is discouraged since it is only available if enabled php.ini file) or short opening tags with echo <?=
(which is always available since PHP 5.4.0 regardless of php.ini file configuration) not removed. The following very short script outputs the value of the variable $temp
:
<?=$temp; ?>
print vs echo command
The difference between print
and echo
is that echo
can output more than one argument:
echo "Hello, ", "world";
The print
and echo
statements are also often seen with parentheses:
echo "hello"; // is the same as echo ("hello");
Parentheses make no difference to the behavior of print
. However, when they are used with echo
, only one output parameter can be provided.
The echo
and print
statements can be used for most tasks and can output any combination of static strings, numbers, arrays, and other variable types discussed later in this tutorial. We discuss more complex output with printf()
, sprintf()
, and fprintf()
later in this section.
In next tutorial, you’ll learn how to create and run a PHP script on your own PC (or laptop).
Getting Started with PHP: