Figure 5-7 illustrates the principle of one-component querying. When the user selects a link on a page-let's assume this page is named browse.php and we refer to this as the calling page-an HTTP request for a PHP script addcart.php is sent to the server. At the server, the script addcart.php is interpreted by the PHP script engine and, after carrying out the database actions in the script, no output is produced. Instead-and this is the key to one-component querying-an HTTP Location:
header is sent as a response to the web browser, and this header causes the browser to request the original calling page, browse.php. The result is that the calling page is redisplayed, and the user has the impression that he remained on the query input component page.
Figure 5-7. The principle of one-component querying
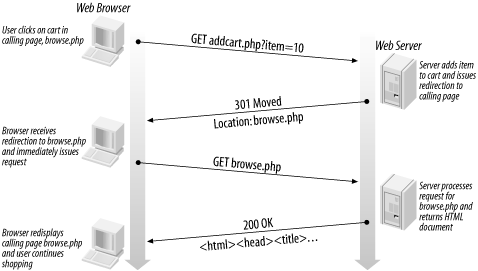
A good example of an application of one-component queryING was illustrated in the last section, where we showed how Add to Cart functionality can be incorporated in the winestore panel. One excellent way to support Add to Cart is to author a script that adds the wine to the user's cart and then redirects the user back to the panel. The cart is updated after a click, and the user can continue reading about and, hopefully, purchasing wines.
Example 5-8 shows a one-component script. In practice, the script adds a quantity of a specific wine to a shopping cart, using the parameters embedded in the links in the page generated by the script in Example 5-7. However, for simplicity we have not included the database queries here; modifying the database is the subject of the next chapter, and the full code for this example is presented in Chapter 11.
Example 5-8. Implementing one-component querying for the Add to Cart functionality
<? if (!empty($wineId) && !empty($qty)) { // Database functionality goes here // This is the key to one-component querying: // Redirect the browser back to the calling page, // using the HTTP response header "Location:" // and the PHP environment variable $HTTP_REFERER header("Location: $HTTP_REFERER"); exit; } else echo "Incorrectly called."; ?>
The key to Example 5-8 is the final two lines of a successful execution of the script:
header("Location: $HTTP_REFERER"); exit;
The header( )
function sends an additional HTTP response header. In one-component querying, the response includes the Location
header that redirects a browser to another URL, in this case the URL of the calling page. The URL of the calling page is automatically initialized into the PHP web server environment variable $HTTP_REFERER
. The exit
statement causes the script to abort after sending the header.
Consider an example where the calling page is the resource example.5-7.php that is output by the script in Example 5-7. This is the page that shows the user the Hot New Wines panel and allows the user to click on a link to add an item to her shopping basket. The user then clicks on a link on this page and requests this URL:
http://localhost/example.5-8.php?qty=1&wineId=801
After successfully completing the request by running the script in Example 5-8 and adding the item to the shopping cart, the following header is sent back to the browser as a response:
Location: http://localhost/example.5-7.php
This header redirects the browser back to the calling page, completing the one-component query.
|
One-component querying is useful in situations where only the query screen is required or the results page and the query page are the same page. For example, in the winestore, one-component querying is used to update quantities in the shopping cart when the user alters the quantities of wine in his shopping cart. In general, one-component querying works well for simple update operations; these are the subject of Chapter 6.