|
Client-side validation is optional but has benefits, including faster response to the user than server-side validation, a reduction in web-server load, and a reduction in network traffic. Moreover, client-side validation can be implemented as interactive validation, not only as post-validation, as on the server side. However, validation in the client tier is unreliable: the user can bypass the validation through design, error, or configuration. For that reason, client-side validation is a tool that should be used only to improve speed, reduce load, and add features, and never to replace server-side validation.
Consider the short JavaScript validation example in Example 7-1.
Example 7-1. A simple JavaScript example to check if a <form> field is empty
<!DOCTYPE HTML PUBLIC "-//W3C//DTD HTML 4.0 Transitional//EN" "http://www.w3.org/TR/html4/loose.dtd"> <html> <head> <title>Simple JavaScript Example</title> <script type="text/javascript"> <!-- Hide the script from old browsers function containsblanks(s) { for(var i = 0; i < s.value.length; i++) { var c = s.value.charAt(i); if ((c == ' ') || (c == '\n') || (c == '\t')) { alert('The field must not contain whitespace'); return false; } } return true; } // end hiding --> </script> </head> <body> <h2>Username Form</h2> <form onSubmit="return(containsblanks(this.userName));" method="post" action="test.php"> <input type="text" name="userName" size=10> <input type="submit" value="SUBMIT"> </form> </body> </html>
This example is designed to check if a userName
field contains whitespace and, if so, to show a dialog box containing an error message to the user. The dialog box is shown in Figure 7-1.
Figure 7-1. The dialog box produced when whitespace is entered in the userName field
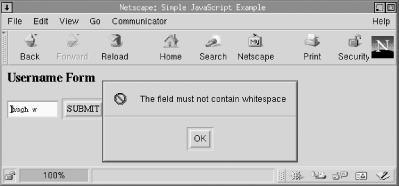
The example contains no PHP but only a mixture of HTML and JavaScript. Almost all the JavaScript is encapsulated between the <script>
and </script>
tags in the first 17 lines of the example. The JavaScript function contained in the tags, containsblanks( )
, is executed when the user submits the <form>
.
The function call is part of the <form>
element:
<form onSubmit="return(containsblanks(this.userName));" method="post" action="test.php">
When the submission event occurs-the user presses the Submit button-the onSubmit
action handler is triggered. In this case, the function containsblanks( )
is called with one parameter, this.userName
. The object this
refers to the <form>
itself and the expression this.userName
refers to the input widget within the <form>
. The function call itself is wrapped in a return( )
expression. The overall result of executing containsblanks( )
is that if the function returns false
, the <form>
isn't submitted to the server; if the function returns true
, the HTTP request proceeds as usual.
The syntax of the JavaScript code is similar to PHP, and to other languages such as C and Java. The function containsblanks( )
works as follows:
-
A
for
loop repeatedly performs actions on the characters entered by the user. The expressions.value.length
refers to the length of the string value entered by the user into theuserName
widget. Thelength
property is one of the predefined properties of thevalue
attribute of the<input>
widget. -
Each character in the string entered by the user is stored in a character variable
c
.s.value.charAt(i)
is again an expression related to the value entered by the user in the<form>
. Thevalue
attribute of the widget has an associated function (or, more correctly, a method) calledcharAt( )
that returns the value of the character at the position passed as a parameter. For example, if the user enterstest
in the widget,s.value.charAt(0)
returnst
, ands.value.charAt(1)
returnse
. -
The
if
statement checks whether the current character is a space, a tab character, or a carriage return. If so, thealert( )
function is called with an error string as a parameter. Thealert( )
function presents a dialog box in the browser that shows the error message and has an OK button, as shown in Figure 7-1. When the user clicks OK, the function returnsfalse
, and the submission process stops. -
If the string doesn't contain any whitespace, the function
containsblanks( )
returnstrue
, and the<form>
submits as usual.
Note that the HTML comment tags are included inside the <script>
tags and surround the actual body of the JavaScript script. This is good practice, because if JavaScript is disabled or the user has an old browser that knows nothing about scripts, the comments hide the script from a potentially confused browser. An old browser happily displays the HTML page as usual, and most also ignore the onSubmit
event handler in the <form>
element.